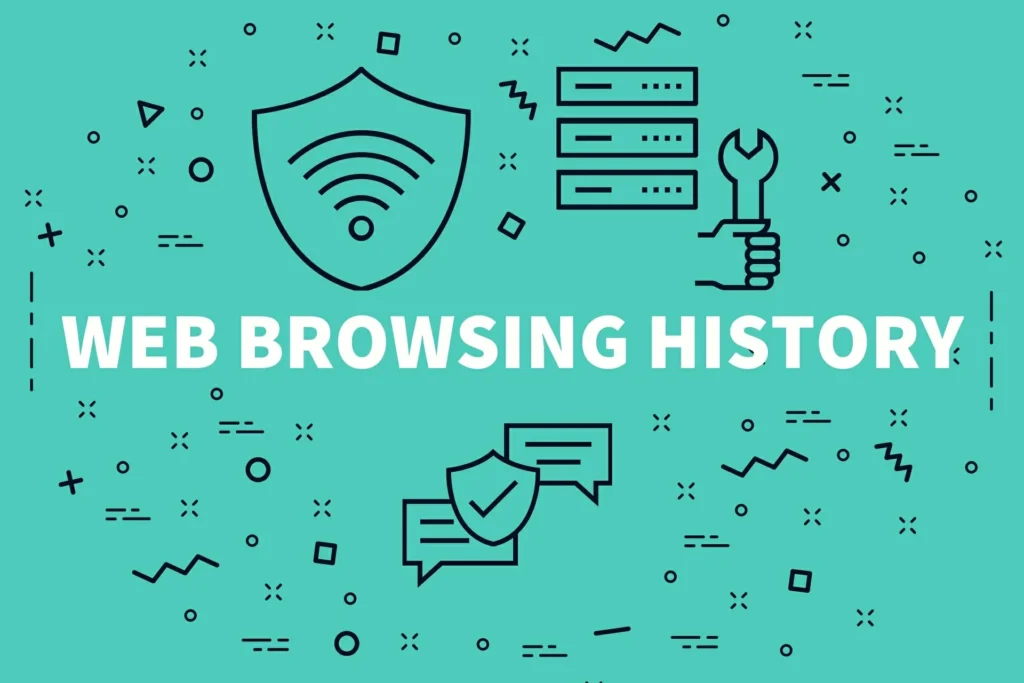
Table of Contents
In this post, I will show you how to extract browsing history from Google Chrome, Mozilla Firefox and Microsoft Edge in Windows operating systems using DB Browser for SQLite and Python.
History file location
# Chrome
C:\Users\<Username>\AppData\Local\Google\Chrome\User Data\Default\History
# Firefox
C:\Users\<Username>\AppData\Roaming\Mozilla\Firefox\Profiles\<ProfileName>\places.sqlite
# Edge
C:\Users\<Username>\AppData\Local\Microsoft\Edge\User Data\Default\History
DB Browser for SQLite
If the browser is currently running, we need to copy the history file to elsewhere to avoid getting the error "database is locked".
File -> Open Database Read Only
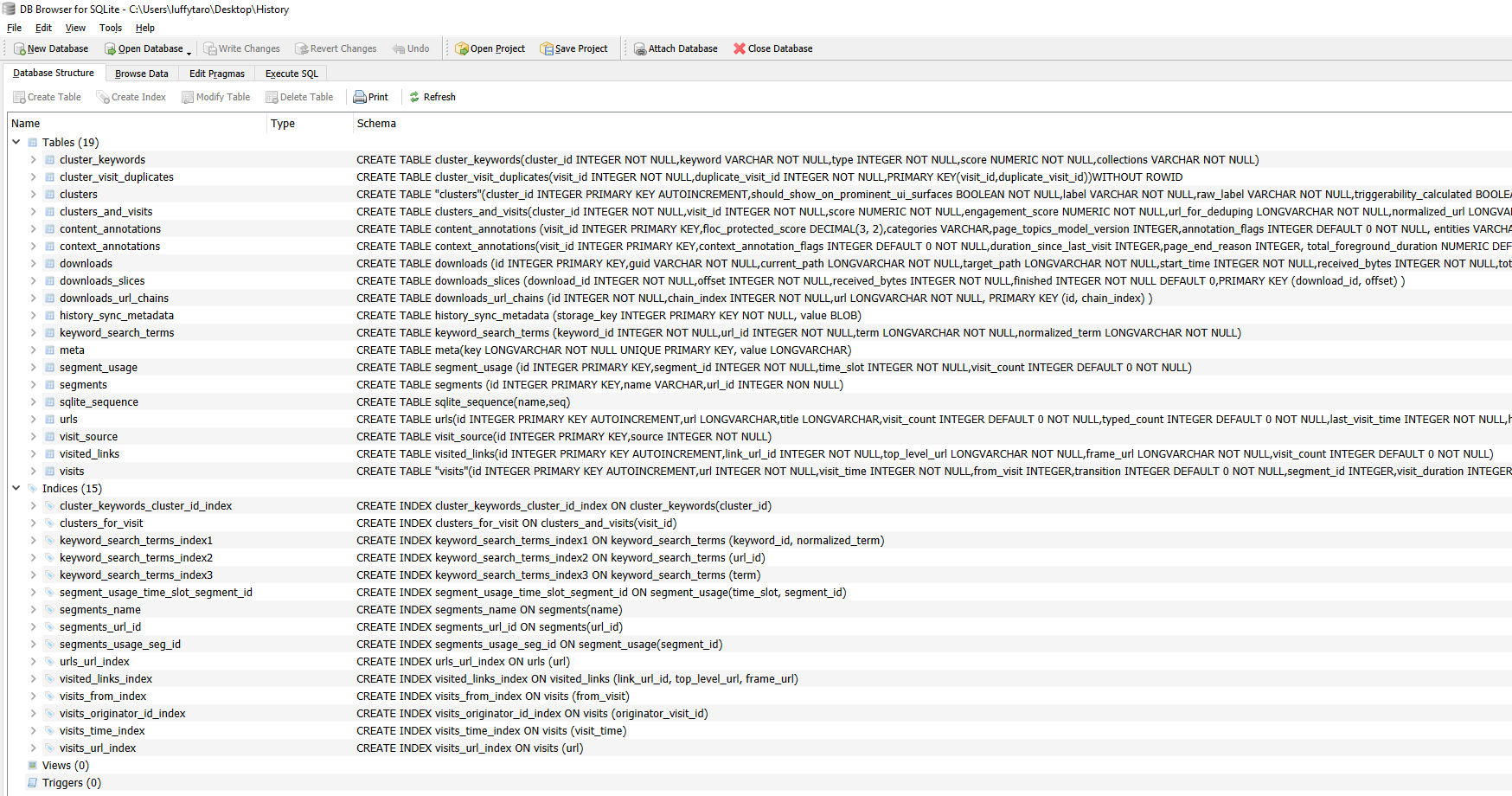
In Chrome, the urls table contains all visited URLs and its timestamps.
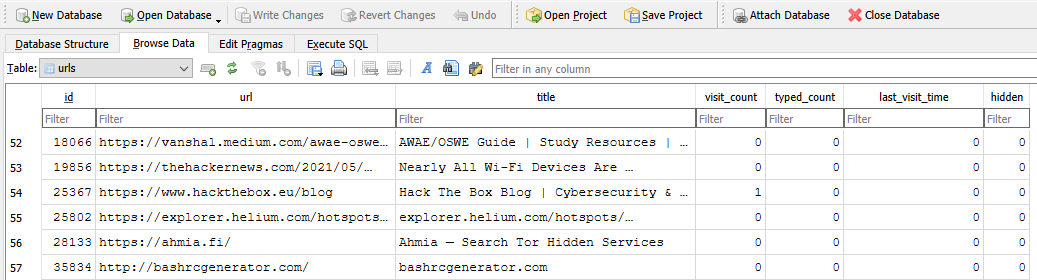
Using custom SQL query for better readability.
-- Chrome & Edge
SELECT
datetime(last_visit_time/1000000-11644473600, "unixepoch") as last_visited,
url,
title,
visit_count
FROM urls ORDER BY last_visit_time DESC;
-- Firefox
SELECT
datetime(moz_historyvisits.visit_date/1000000,'unixepoch'),
moz_places.url,
moz_places.title
FROM
moz_places,
moz_historyvisits
WHERE
moz_places.id = moz_historyvisits.place_id;
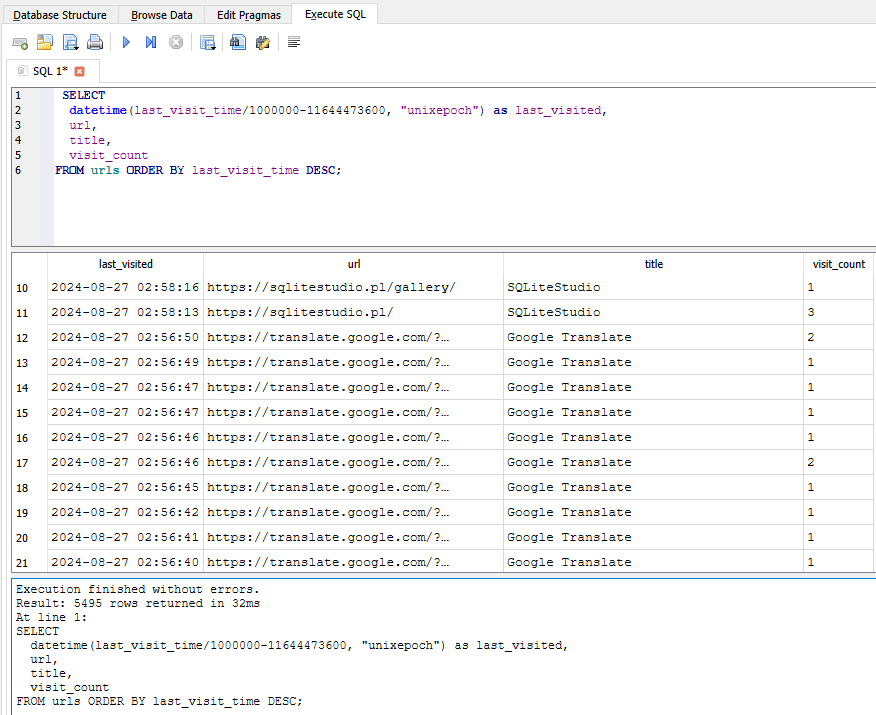
Python Implementation
The following Python script will export the specific browsing history into a csv file.
import sqlite3
import csv
import os
# Define the path to the History file
history_path = r"C:\Users\<YourUsername>\AppData\Local\Google\Chrome\User Data\Default\History"
conn = sqlite3.connect(history_path)
cursor = conn.cursor()
cursor.execute("SELECT datetime(last_visit_time/1000000-11644473600, 'unixepoch') as last_visited, url, title, visit_count FROM urls ORDER BY last_visit_time DESC;")
rows = cursor.fetchall()
output_csv_path = "history_urls.csv"
# Write the data to a CSV file
with open(output_csv_path, "w", newline='', encoding="utf-8") as csvfile:
csvwriter = csv.writer(csvfile)
csvwriter.writerow(["last_visit", "url", "title", "visit_count"])
csvwriter.writerows(rows)
conn.close()
print(f"Data exported successfully to {output_csv_path}")